Descriptors
All USB devices have a hierarchy of descriptors that describe various features of the device: the manufacturer ID, the version of the device, the version of USB it supports, what the device is, its power requirements, the number and type of endpoints, and so forth.
The most common USB descriptors are:
• Device descriptors
• Configuration descriptors
• Interface descriptors
• HID descriptors
• Endpoint descriptors
The descriptors are in a hierarchical structure as shown in Figure 8.7. At the top of the hierarchy we have the device descriptor, then the configuration descriptors, followed by the interface descriptors, and finally the endpoint descriptors. The HID descriptor always follows the interface descriptor when the interface belongs to the HID class.
All descriptors have a common format. The first byte (bLength) specifies the length of the descriptor, while the second byte (bDescriptorType) indicates the descriptor type.
Device Descriptors
The device descriptor is the top-level set of information read from a device and the first item the host attempts to retrieve.
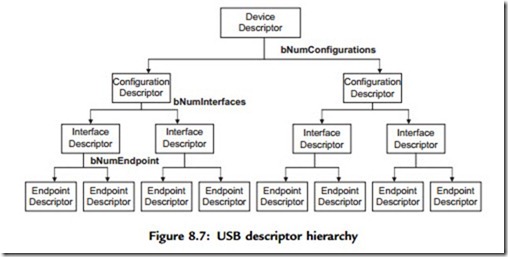
A USB device has only one device descriptor, since the device descriptor represents the entire device. It provides general information such as manufacturer, serial number, product number, the class of the device, and the number of configurations.
Table 8.5 shows the format for a device descriptor with the meaning of each field.
bLength is the length of the device descriptor.
bDescriptorType is the descriptor type.
bcdUSB reports the highest version of USB the device supports in BCD format. The number is represented as 0xJJMN, where JJ is the major version number, M is the minor version number, and N is the subminor version number. For example, USB 1.1 is reported as 0x0110.
bDeviceClass, bDeviceSubClass, and bDeviceProtocol are assigned by the USB organization and are used by the system to find a class driver for the device.
bMaxPacketSize0 is the maximum input and output packet size for endpoint 0. idVendor is assigned by the USB organization and is the vendor’s ID. idProduct is assigned by the manufacturer and is the product ID.
bcdDevice is the device release number and has the same format as the bcdUSB.
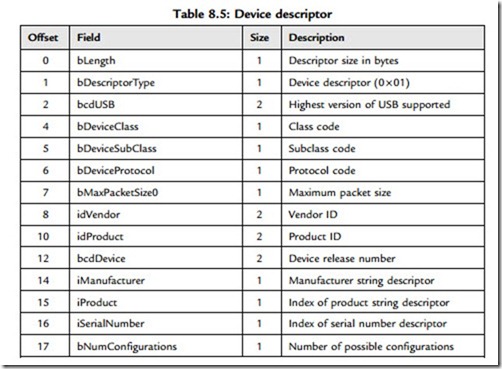
iManufacturer, iProduct, and iSerialNumber are details about the manufacturer and the product. These fields have no requirement and can be set to zero.
bNumConfigurations is the number of configurations the device supports.
Table 8.6 shows an example device descriptor for a mouse device. The length of the descriptor is 18 bytes (bLength ¼ 18), and the descriptor type is 0x01 (bDescriptorType
¼ 0x01). The device supports USB 1.1 (bcdUSB ¼ 0x0110). bDeviceClass, bDeviceSubClass, and bDeviceProtocol are set to zero to show that the class information is in the interface descriptor. bMaxPacketSize0 is set to 8 to show that the maximum input and output packet size for endpoint 0 is 8 bytes. The next three bytes identify the device by the vendor ID, product ID, and device version number. The next three items define indexes to strings about the manufacturer, product, and the serial number. Finally, we notice that the mouse device has just one configuration (bNumConfigurations ¼ 1).

Configuration Descriptors
The configuration descriptor provides information about the power requirements of the device and how many different interfaces it supports. There may be more than one configuration for a device.
Table 8.7 shows the format of the configuration descriptor with the meaning of each field.
bLength is the length of the device descriptor.
bDescriptorType is the descriptor type.
wTotalLength is the total combined size of this set of descriptors (i.e., total of configuration descriptor þ interface descriptor þ HID descriptor þ endpoint descriptor). When the configuration descriptor is read by the host, it returns the entire configuration information, which includes all interface and endpoint descriptors.
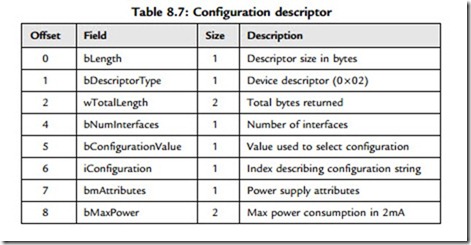
bNumInterfaces is the number of interfaces present for this configuration.
bConfigurationValue is used by the host (in command SetConfiguration) to select the configuration.
iConfiguration is an index to a string descriptor describing the configuration in readable format.
bmAttributes describes the power requirements of the device. If the device is USB bus-powered, then bit D7 is set. If it is self-powered, it sets bit D6. Bit D5 specifies the remote wakeup of the device. Bits D7 and D0–D4 are reserved.
bMaxPower defines the maximum power the device will draw from the bus in 2mA units.
Table 8.8 shows an example configuration descriptor for a mouse device. The length of the descriptor is 9 bytes (bLength ¼ 9), and the descriptor type is 0x02 (bDescriptorType ¼ 0x02). The total combined size of the descriptors is 34 (wTotalLength ¼ 34). The number of interfaces for the mouse device is 1 (bNumInterfaces ¼ 1). Host SetConfiguration command must use the value 1 as an argument in SetConfiguration() to select this configuration. There is no string to describe this configuration. bmAttributes is set to 0x40 to indicate that the device is self-powered. bMaxPower is set to 10 to specify that the maximum current drawn by the device is 20mA.

bLength is the length of the device descriptor.
bDescriptorType is the descriptor type.
bInterfaceNumber indicates the index of the interface descriptor.
bAlternateSetting can be used to specify alternate interfaces that can be selected by the host using command Set Interface.
bNumEndpoints indicates the number of endpoints used by the interface. bInterfaceClass specifies the device class code (assigned by the USB organization). bInterfaceSubClass specifies the device subclass code (assigned by the USB organization).
bInterfaceProtocol specifies the device protocol code (assigned by the USB organization).
iInterface is an index to a string descriptor of the interface.
Table 8.10 shows an example interface descriptor for a mouse device. The descriptor length is 9 bytes (bLength ¼ 9) and the descriptor type is 0x04 (bDescriptorType ¼ 0x04). The interface number used to reference this interface is 1 (bInterfaceNumber ¼ 1).
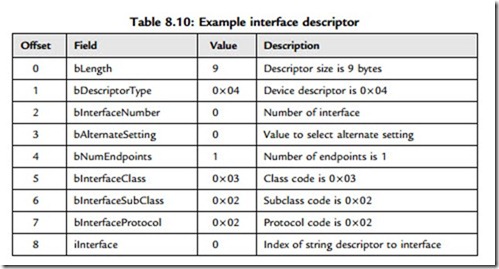
bAlternateSetting is set to 0 (i.e., no alternate interfaces). The number of endpoints used by this interface is 1 (excluding endpoint 0), and this is the endpoint used for the mouse to send its data. The device class code is 0x03 (bInterfaceClass ¼ 0x03). This is an HID (human interface device) type class. The interface subclass is set to 0x02. The device protocol is 0x02 (mouse). There is no string to describe this interface (iInterface ¼ 0).
HID Descriptors
An HID descriptor always follows an interface descriptor when the interface belongs to the HID class. Table 8.11 shows the format of the HID descriptor.
bLength is the length of the device descriptor. bDescriptorType is the descriptor type. bcdHID is the HID class specification.
bCountryCode specifies any special local changes.
bNumDescriptors specifes if there are any additional descriptors associated with this class.
bDescriptorType is the type of the additional descriptor specified in
bNumDescriptors.
wDescriptorLength is the length of the additional descriptor in bytes.

Table 8.12 shows an example HID descriptor for a mouse device. The length of the descriptor is 9 bytes (bLength ¼ 9), and the descriptor type is 0x21 (bDescriptorType
¼ 0x21). The HID class is set to 1.1 (bcdHID ¼ 0x0110). The country code is set to zero (bCountryCode ¼ 0), specifying that there is no special localization with this device. The number of descriptors is set to 1 (bNumDescriptors ¼ 1) which specifies that there is one additional descriptor associated with this class. The type of the additional descriptor is REPORT (bDescriptorType ¼ REPORT), and its length is 52 bytes (wDescriptorLength ¼ 52).
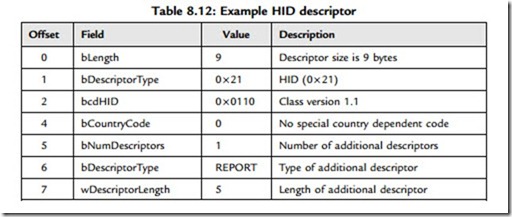
Endpoint Descriptors
Table 8.13 shows the format of the endpoint descriptor. bLength is the length of the device descriptor. bDescriptorType is the descriptor type. bEndpointAddress is the address of the endpoint. bmAttributes specifies what type of endpoint it is. wMaxPacketSize is the maximum packet size.
bInterval specifies how often the endpoint should be polled (in ms).
Table 8.14 shows an example endpoint descriptor for a mouse device. The length of the descriptor is 7 bytes (bLength ¼ 7), and the descriptor type is 0x05 (bDescriptorType
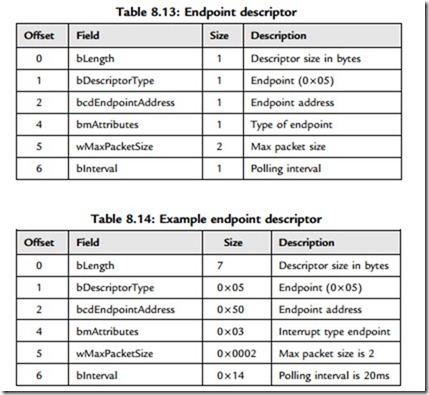
¼ 0x05). The endpoint address is 0x50 (bEndpointAddress ¼ 0x50). The endpoint is to be used as an interrupt endpoint (bmAttributes ¼ 0x03). The maximum packet size is set to 2 (wMaxPacketSize ¼ 0x02) to indicate that packets longer than 2 bytes will not be sent from the endpoint. The endpoint should be polled at least once every 20ms (bInterval ¼ 0x14).