PIC18F258 Microcontroller
Later in this chapter the PIC18F258 microcontroller is used in a CAN bus–based project. This section describes this microcontroller and its operating principles with respect to its built-in CAN bus. The principles here are in general applicable to other PIC microcontrollers with CAN modules.
The PIC18F258 is a high performance 8-bit microcontroller with integrated CAN module. The device has the following features:
• 32K flash program memory
• 1536 bytes RAM data memory
• 256 bytes EEPROM memory
• 22 I/O ports
• 5-channel 10-bit A/D converters
• Three timers/counters
• Three external interrupt pins
• High-current (25mA) sink/source
• Capture/compare/PWM module
• SPI/I2C module
• CAN 2.0A/B module
• Power-on reset and power-on timer
• Watchdog timer
• Priority level interrupts
• DC to 40MHz clock input
• 8 x 8 hardware multiplier
• Wide operating voltage (2.0V to 5.5V)
• Power-saving sleep mode
The features of the PIC18F258 microcontroller’s CAN module are as follows:
• Compatible with CAN 1.2, CAN 2.0A, and CAN 2.0B
• Supports standard and extended data frames
• Programmable bit rate up to 1Mbit/s
• Double-buffered receiver
• Three transmit buffers
• Two receive buffers
• Programmable clock source
• Six acceptance filters
• Two acceptance filter masks
• Loop-back mode for self-testing
• Low-power sleep mode
• Interrupt capabilities
The CAN module uses port pins RB3/CANRX and RB2/CANTX for CAN bus receive and transmit functions respectively. These pins are connected to the CAN bus via an MCP2551-type CAN bus transceiver chip.
The PIC18F258 microcontroller supports the following frame types:
• Standard data frame
• Extended data frame
• Remote frame
• Error frame
• Overload frame
• Interframe space
A node uses filters to decide whether or not to accept a received message. Message filtering is applied to the whole identifier field, and mask registers are used to specify which bits in the identifier the filters should examine.
The CAN module in the PIC18F258 microcontroller has six modes of operation:
• Configuration mode
• Disable mode
• Normal operation mode
• Listen-only mode
• Loop-back mode
• Error recognition mode
Configuration Mode
The CAN module is initialized in configuration mode. The module is not allowed to enter configuration mode while a transmission is taking place. In configuration mode the module will neither transmit nor receive, the error counters are cleared, and the interrupt flags remain unchanged.
Disable Mode
In disable mode, the module will neither transmit nor receive. In this mode the internal clock is stopped unless the module is active. If the module is active, it will wait for 11 recessive bits on the CAN bus, detect that condition as an IDLE bus, and then accept the module disable command. The WAKIF interrupt (wake-up interrupt) is the only CAN module interrupt that is active in disable mode.
Normal Operation Mode
The normal operation mode is the CAN module’s standard operating mode. In this mode, the module monitors all bus messages and generates acknowledge bits, error frames, etc. This is the only mode that can transmit messages.
Listen-only Mode
The listen-only mode allows the CAN module to receive messages, including messages with errors. It can be used to monitor bus activities or to detect the baud rate on the bus. For automatic baud rate detection, at least two other nodes must be communicating with each other. The baud rate can be determined by testing different values until valid messages are received. The listen-only mode cannot transmit messages.
Loop-Back Mode
In the loop-back mode, messages can be directed from internal transmit buffers to receive buffers without actually transmitting messages on the CAN bus. This mode is useful during system developing and testing.
Error Recognition Mode
The error recognition mode is used to ignore all errors and receive all messages. In this mode, all messages, valid or invalid are received and copied to the receive buffer.
CAN Message Transmission
The PIC18F258 microcontroller implements three dedicated transmit buffers: TXB0, TXB1, and TXB2. Pending transmittable messages are in a priority queue. Before the SOF is sent, the priorities of all buffers queued for transmission are compared. The transmit buffer with the highest priority is sent first. If two buffers have the same priority, the one with the higher buffer number is sent first. There are four levels of priority.
CAN Message Reception
Reception of a message is a more complex process. The PIC18F258 microcontroller includes two receive buffers, RXB0 and RXB1, with multiple acceptance filters
for each (see Figure 9.13). All received messages are assembled in the message assembly buffer (MAB). Once a message is received, regardless of the type of identifier and the number of data bytes, the entire message is copied into the MAB.
Received messages have priorities. RXB0 is the higher priority buffer, and it has two message acceptance filters, RXF0 and RXF1. RXB1 is the lower priority buffer and has four acceptance filters: RXF2, RXF3, RXF4, and RXF5. Two programmable acceptance filter masks, RXM0 and RXM1, are also available, one for each receive buffer.
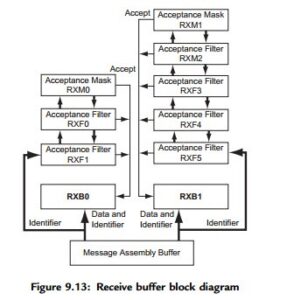
The CAN module uses message acceptance filters and masks to determine if a message in the MAB should be loaded into a receive buffer. Once a valid message is received by the MAB, the identifier field of the message is compared to the filter values. If there is a match, that message is loaded into the appropriate receive buffer. The filter masks determine which bits in the identifier are examined with the filters. The truth table in Table 9.3 shows how each bit in the identifier is compared against
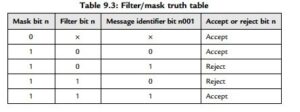
the masks and filters to determine if the message should be accepted. If a mask bit is set to 0, that bit in the identifier is automatically accepted regardless of the filter bit.
Calculating the Timing Parameters
Setting the nodes’ timing parameters is essential for the bus to operate reliably. Given the microcontroller clock frequency and the required CAN bus bit rate, we can calculate the values of the following timing parameters:
• Baud rate prescaler value
• Prop_Seg value
• Phase_Seg1 value
• Phase_Seg2 value
• SJW value
Correct timing requires that
• Prop_Seg þ Phase_Seg1 ::: Phase_Seg2
• Phase_Seg2 ::: SJW
The following example illustrates the calculation of these timing parameters.
Example 9.2
Assuming the microcontroller oscillator clock rate is 20MHz and the required CAN bit rate is 125KHz, calculate the timing parameters.
Solution 9.2
With a 20MHz clock rate, the clock period is 50ns. Choosing a baud rate prescaler value of 4, from Equation (9.4), TQ ¼ 2 * (BRP þ 1) * TOSC, gives a time quantum of TQ ¼ 500ns. To obtain a nominal bit rate of 125KHz, the nominal bit time must be:
TBIT ¼ 1=0:125MHz ¼ 8ms; or 16TQ
The Sync_Segment is 1TQ. Choosing 2TQ for the Prop_Seg, and 7TQ for Phase_Seg1 leaves 6TQ for Phase_Seg2 and places the sampling point at 10TQ at the end of Phase_Seg1.
By the rules described earlier, the SJW can be the maximum allowed (i.e., 4). However, a large SJW is only necessary when the clock generation of different nodes is not stable or accurate (e.g., if ceramic resonators are used). Typically, a SJW of 1 is enough. In summary, the required timing parameters are:
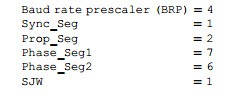
The sampling point is at 10TQ which corresponds to 62.5% of the total bit time.
There are several tools available for free on the Internet for calculating CAN bus timing parameters. One such tool is the CAN Baud Rate Calculator, developed by Artic Consultants Ltd (http://www.articconsultants.co.uk). An example using this tool follows.
Example 9.3
Assuming the microcontroller oscillator clock rate is 20MHz and the required CAN bit rate is 125KHz, calculate the timing parameters using the CAN Baud Rate Calculator.
Solution 9.3
Figure 9.14 shows the output of the CAN Baud Rate Calculator program. The device type is selected as PIC18Fxxx8, the oscillator frequency is entered as 20MHz, and the CAN bus baud rate is entered as 125KHz.
Clicking the Calculate Settings button calculates and displays the recommended timing parameters. In general, there is more than one solution, and different solutions are given in the Calculated Solutions field’s drop-down menu.
In choosing Solution 2 from the drop-down menu, the following timing parameters are recommended by the program:
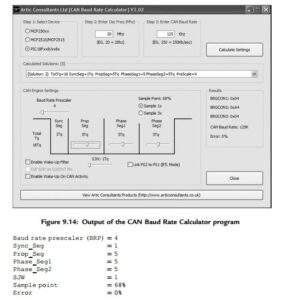