BANK1 QUALIFIER
The bank1 type qualifier is used to store static variables in RAM bank 1 of the microcontroller. By default, variables are stored in bank 0 of the RAM. In the following example, the static integer variable temp is stored in bank 1:
static bank1 int temp;
ARRAYS
An array is a collection of variables of the same type. For example, an integer array called
results and consisting of 10 elements is declared as follows:
int results[10];
As shown in Figure 4.1, each array element has an index and the indices starts at 0. In this example, the first element has index 0 and the last element has index 9. An element of the array is accessed by specifying the index of the required element in a square bracket. For example, the first element of the array is results[0], the second element is results[1], and the last element is results[9]. Any element of an array can be assigned a value which is same type as the type of the array. For example, the integer value 25 can be assigned to the second element of the above array as:
results[1] = 25;
Initial values can be assigned to all elements of an array by separating the values by commas and enclosing them in a curly bracket. The size of the array should not be specified in such declarations. In the following example, odd numbers are assigned to the elements of array results and the size of this array is set automatically to 10:
int results[] = {1, 3, 5, 7, 9, 11, 13, 15, 17, 19};
The elements of an array can be stored in either the RAM memory or the program memory of a PIC microcontroller. If the array elements never change in a program then they should be stored in the program memory so that the valuable RAM locations can be used for other purposes. Array elements are stored in the program memory if the array name is preceded by the keyword const. In the following example, it is assumed that the array elements never change in a program and thus they are stored in the program memory of the microcontroller:
const int results[] = {1, 3, 5, 7, 9, 11, 13, 15, 17, 19};
Arrays can also store characters. In the following example, the characters COMPUTER is stored in a character array called device:

In this example, the size of array device is set to 8 and the elements of this array are:
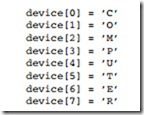
Another way of storing characters in an array is as string variables. Strings are a collection of characters stored in an array and terminated with the null character (ASCII 0). For example, the characters COMPUTER can be stored as a string in array device as:
unsigned char device[] = ’COMPUTER’;
In this example, the array size is set to 9 and the last element of the array is the null character,
i.e. the elements of array device are:
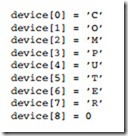
Arrays can have multiple dimensions. For example, a two-dimensional array is declared by specifying the size of its rows followed by the size of its columns. In the example below, a two-dimensional integer array having three rows and five columns is declared. The array is given the name temp:
int temp[3][5];
This array has a total of 15 elements as shown in Figure 4.2. The first element (top left-hand) is indexed as temp[0][0] and the last element (bottom-right) is indexed as temp[2][4]. Values can be loaded into the array elements by specifying the row and the column index of the location to be loaded. For example, integer number 50 is loaded into the first row and third column of array temp:
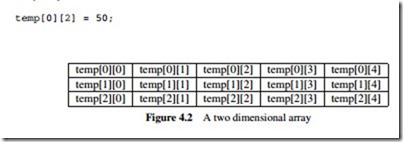