FUNCTIONS IN C
Almost all programming languages support functions or some similar concepts. Some lan- guages call them subroutines, some call them procedures. Some languages distinguish between functions which return variables and those which do not.
In almost all programming languages functions are of two kinds: user functions and built-in functions. User functions are developed by programmers, while built-in functions are usually general purpose routines provided with the compiler. Functions are independent program codes and are usually used to return values to the main calling programs.
In this section we shall look at both types of functions.
User Functions
These functions are developed by the programmer. Every function has a name and optional arguments, and a pair of brackets must be used after the function name in order to declare the arguments. The function performs the required operation and can return values to the main calling program if required. Not all functions return values. Functions whose names start with the keyword void do not return any values, as shown in the following example:
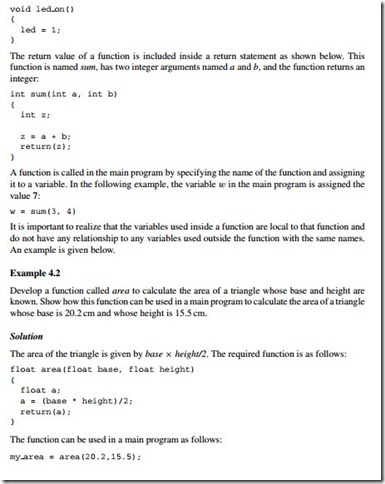
Built-in Functions
The PICC Lite compiler provides a large number of built-in functions which are available to the programmer. A list of all the available functions can be obtained from the PICC Lite User’s Guide. Some examples are given below.
abs
This function calculates the absolute value of a given number. For example, the absolute value of the variable total can be obtained as follows:

cos
This function returns the trigonometric cosine of an angle. The angle must be in radians. For example, the cosine of 45◦ can be calculated as follows:
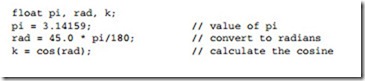
sqrt
The sqrt function returns the square root of a given number. In the following example, the square root of number 14.5 is stored in the variable no:
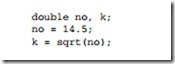
isupper
This function checks whether or not a given character is upper case between A and Z. If it is, a 1 is returned, otherwisea0 is returned. An example is given below:

isalnum
This function checks whether or not a given character is an alphanumeric character between 0 and 9, a and z, or A and Z. An example is given below:
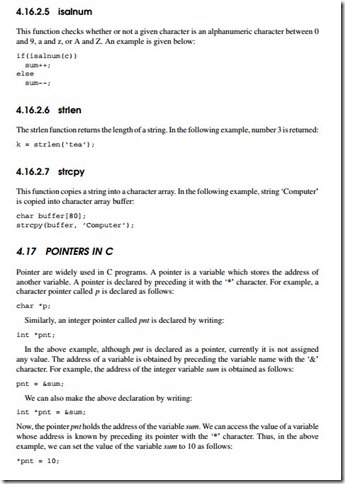